Common Language Runtime (CLR) Explained: Core of .NET
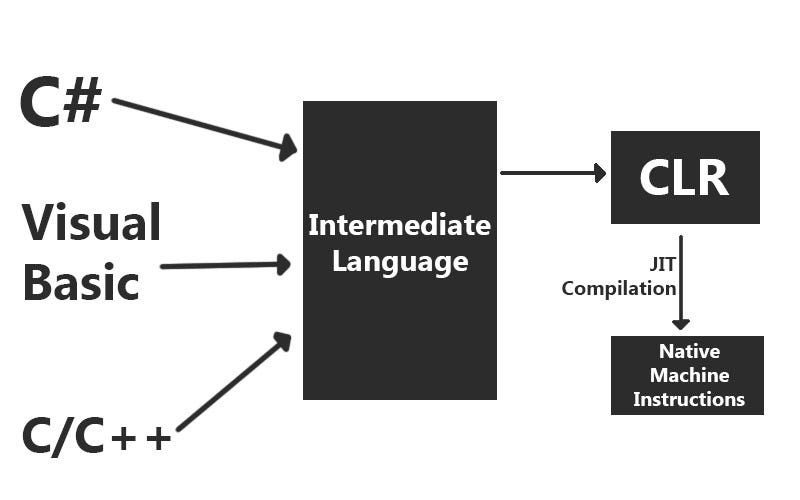
The Common Language Runtime, or CLR, is the virtual execution engine at the heart of Microsoft’s .NET Framework. All .NET programs run under the CLR’s supervision, which provides core services like memory management, security, exception handling and debugging support.
Understanding the CLR is key to understanding the fundamentals of the .NET programming model. In this comprehensive guide, we will dig into how the CLR works and its vital role in .NET applications.
The CLR can be thought of as a “virtual operating system” for .NET code. While programs targeting the CLR run atop the underlying Windows OS, the CLR provides an additional layer abstracting away direct OS calls. Key responsibilities include:
- Memory allocation and management
- Thread scheduling and synchronization
- Code verification for security
- Exception handling
- Garbage collection
- Debugging support
The runtime aims to provide these services reliably and consistently regardless of the programming language used. The CLR’s componentized structure also allows enhancements over time without requiring language changes.
Microsoft positions the CLR as fulfilling “the promise of component-based programming models” by providing a robust, secure execution container.
Execution Model
The key reason the CLR can provide language interoperability is via its compiled intermediate language. Source code from .NET programming languages like C# does not compile directly into OS executable instructions. Instead, it compiles to Common Intermediate Language (CIL) bytecode packaged into a portable executable (PE) format.
When executed, the CLR’s just-in-time (JIT) compiler translates this CIL into native CPU instructions just before execution. This provides portability while enabling optimizations based on the runtime environment. The CLR determines appropriate optimizations such as threading, object allocation, and code in lining.
In the dynamic landscape of web development, harnessing the power of the CLR becomes even more significant. A proficient web development company utilizes the capabilities of .NET and the CLR to build scalable, secure, and performance-driven web applications. By leveraging language interoperability, portability, and runtime optimizations, such companies can deliver robust solutions that meet the evolving demands of the digital world.
So, whether you are a developer exploring the intricacies of the CLR or a business seeking a reliable web development partner, understanding how the CLR facilitates language interoperability and optimizations is crucial. In the hands of a skilled web development company, the synergy of .NET and CLR opens doors to innovative and efficient web solutions.
Common Language Infrastructure
The CLR is part of a larger specification called the Common Language Infrastructure (CLI). The CLI outlines several components:
- Common Type System– This defines how types are declared, used, and managed
- Metadata– Data about .NET programs including assemblies, types, and versions
- Common Intermediate Language– Platform agnostic instruction set
- Common Language Specification– Generic interfaces for language interoperability
These specifications allow seamless interaction between languages and libraries under the managed .NET environment.
Just-In-Time (JIT) Compilation
As mentioned above, the CLR utilizes a just-in-time compiler to translate CIL bytecode into native CPU instructions at runtime right before execution. This provides portability across platforms and optimizes real-time environment data and processor instruction sets.
The Windows OS provides multiple JIT compilers within the CLR in order to support various CPU architectures. For example, there are separate JIT compilers for 32-bit x86, 64-bit AMD64 and ARM chips. The appropriate compiler is invoked based on the executing environment.
Just-in-time compilation introduces a modest performance delay – code executing for the first time incurs this translation cost. But subsequently executed code runs at full native speed, benefiting from prior JIT operations and hot code optimizations. Overall, the flexibility of just-in-time compilation generates faster-running code optimized for the specific system environment.
Memory Management
Memory management is one of the most important services provided by the CLR. It automatically allocates and freeing up objects and variables used by .NET applications. When a .NET program requests memory via `new` or other constructs, the CLR’s memory manager allocates the needed space from the managed heap. When the program no longer references an object, the CLR deallocates the memory, making it available for future allocations.
This automated process prevents memory leaks that can crash long running applications. The CLR uses a high-performance garbage collector that operates automatically, freeing developers from manual memory management.
The garbage collector runs periodically performing several duties:
- Identifying objects no longer referenced
- Reclaiming unused memory
- Defragmenting memory to optimize allocation
- Handling variables that escape scope
The CLR also provides managed objects that abstract unmanaged memory, such as arrays, strings, and containers. This provides access to native memory management only when necessary.
Common Type System
The Common Type System describes how the CLR declares, uses, and manages types. This enables cross-language compatibility with a consistent system across .NET languages.
Some key aspects include:
- Supports classes, interfaces, Enum’s, delegates etc.
- Classes are reference types allocated on the heap
- Structs are value types allocated on thread stacks
- Interface-based programming model
- Single inheritance for implementation
- Multiple interface inheritance
The CTS provides consistent rules enforced by the CLR across .NET languages. This allows values and objects to flow between languages seamlessly at runtime.
Security
As a core focus, the CLR provides several security mechanisms:
Code Access Security
Code access security (CAS) allows controlling what managed code is allowed to do based on defined policies. For example, restricting file or network access. Security permissions are defined in code using attributes or via configuration. Checks are performed at runtime before executing security sensitive operations. CAS prevents untrusted code from performing protected actions without approval. Permissions can be declared broadly across applications or very granularly within specific methods.
Application Domains
Application domains provide isolation boundaries between executing app suites. If an untrusted DLL fails, it only compromises its own app domain while others remain unaffected. This partitions applications logically even when running in the same process physically.
Code Verification
The CLR verifies code upon execution to ensure type safety, proper memory handling, security permissions and other guarantees. This prevents malicious code from circumventing protections. Only verified code meeting the runtime’s standards is allowed to execute under the CLR.
Role-Based Security
.NET provides role-based security (RBAC) features for authentication and authorization based on user roles. APIs enforce declarative role checks at runtime.
Exception Handling
The CLR provides structured exception handling to propagate and manage errors gracefully:
- Detecting exceptional conditions
- Unwinding stack execution to starting point
- Passing exception to calling code
This allows recovering from errors smoothly or terminating safely when needed. Exceptions can be thrown and caught at different levels, with ultimate control over handling. Uncaught exceptions bubble up to the entry point and can be logged before termination. The call stack also captures exception origin, method calls involved and parameter values – invaluable debugging context. .NET exception handling contrasts sharply with the complex error management required in C programs.
Multithreading
The CLR has built-in support for multithreaded code execution. The threading model is directly exposed to .NET developers via classes like `Thread` and `Monitor`.
Some key capabilities provided:
- Creating and managing threads
- Scheduling thread execution
- Synchronization via locks/mutexes
- Thread pooling to limit creation overhead
- Asynchronous programming APIs
- Atomic operations for thread safety
Robust threading prevents issues like race conditions, deadlocks, and resource contention. To leverage the multithreading hire .NET developers with experience in multithreading.
Garbage Collection
We touched on garbage collection previously – it deserves a deeper look given the benefits provided:
Automatic memory reclamation – Developers don’t manually allocate/release memory. Objects no longer referenced are automatically cleaned up.
Reduced memory leaks – Bugs from incorrect manual memory management are avoided.
Increased developer productivity – Time not spent on manual memory management.
Memory compacting to reduce fragmentation – Heap memory is optimized periodically.
Separate heaps per application domain – Isolates apps for stability.
Generational collection – new objects are collected more frequently.
Lazy collection – Objects that escape collection initially are cleaned up later.
Weak references – Allow references to objects without preventing collection.
These capabilities powerfully combine to lower memory consumption while increasing developer productivity and program stability.
Debugging
Rich debugging support is another key CLR capability. The runtime provides diagnostics information and tooling integration to debug managed code:
- Generate debug symbols and enable debugging via attributes
- Interop with debugger tools like concordance tables
- Provide stack unwinding information
- Integrate debugging services with IDEs
- Diagnostic logging and tracing
Debugging enables analyzing code flow, variables values, resource usage and performance. The CLR provides robust context to debug even the most complex scenarios across languages. The debugging APIs also support customization for specific project needs.
Interoperability
Interoperability with native code is another benefit provided by the CLR. This allows combining managed .NET code with existing native libraries and components:
- Call native code from managed code
- Host the CLR inside unmanaged native apps
- Expose CLR components to COM clients
- Invoke for calling Windows API functions
- COM interop via RCW and CCW
- C++ Interop Support
This interop enables gradually migrating legacy codebases to .NET incrementally. Apps can utilize managed and unmanaged code together.
Open Source CLR
As part of Microsoft’s shift to open source, the .NET runtime including the CLR is open source and available on GitHub:
https://github.com/dotnet/runtime
The CLR source code enables deeper understanding of the inner workings from Microsoft engineers. The codebase also continues accepting contributions to improve the runtime foundations.
The CLR Moving Forward
The CLR has continued evolving since its 1.0 debut in 2002 alongside the rapid innovation across the .NET ecosystem:
- Faster JIT compilation
- AOT (ahead-of-time) compilation support
- SIMD vectorization
- Dynamic code generation
- Improved GC performance
- Linux/MacOS support
- Docker containers
- Expanded APIs and language features
The CLR will continue adapting to leverage operating system and hardware advancements on an ongoing basis. Microsoft also continues tweaking the core virtual machine for greater performance and capabilities. The innovations delivered via the CLR in turn empower all .NET developers.
Summary
The Common Language Runtime sits at the foundation of .NET, providing core execution services consistently across languages and platforms. Understanding the CLR is critical to understanding modern .NET:
- It manages code execution for the managed .NET environment.
- Enables language interoperability via IL bytecode.
- Provides memory management, threading, security and more.
- Optimizes performance via JIT compilation.
- Standardizes types and objects via the CTS.
- Designed for portability and robustness.
- Integrates with debuggers and native code.
- Is evolving to support modern workloads.
With the CLR abstracting the nitty gritty os interactions, developers enjoy great productivity building feature rich .NET applications with peace of mind. The next 20 years of .NET innovation will continue building on the sturdy virtual machine foundation established by the pioneering CLR.